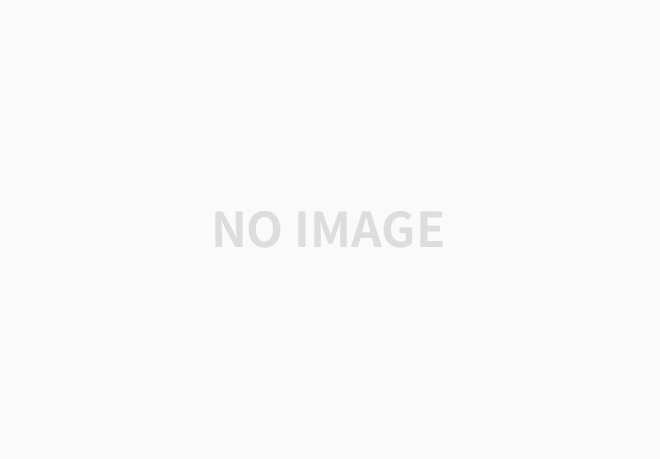
package object;
class Student {
int studentNum;
String studentName;
public Student(int studentNum, String studentName) {
this.studentName = studentName;
this.studentNum = studentNum;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Student) {
Student std = (Student) obj;
return(this.studentNum == std.studentNum);
}
return false;
}
}
public class EqualsTest {
public static void main(String[] args) {
// String str1 = new String("abc");
// String str2 = new String("abc");
//
// System.out.println(str1 == str2);
// System.out.println(str1.equals(str2));
Student Lee = new Student(100, "이순신");
Student Shin = new Student(100, "이순신");
Student Lee2 = Lee;
System.out.println(Lee == Shin);
System.out.println(Lee.equals(Shin));
/*
* false
true
*/
}
}
43. Object 클래스 - 1
package object;
class Book {
String title;
String author;
public Book(String title, String author) {
this.author = author;
this.title = title;
}
@Override // Object 에서 오버라이드
public String toString() {
// TODO Auto-generated method stub
return author + title;
}
}
public class ToStringTest {
public static void main(String[] args) {
Book book = new Book("토지", "박경리");
System.out.println(book);
String str = new String("토지");
System.out.println(str);
System.out.println(str.toString());
}
}
| Object 클래스
모든 클래스의 최상위 클래스
java.lang.Object 클래스
모든 클래스는 Object 클래스에서 상속을 받음
모든 클래스는 Object 클래스에 메서드를 사용할 수 있음.
모든 클래스는 Object 클래스에 일부 메서드를 재정의 하여 사용할 수 있음(final 은 재정의가 안된다.)
44. Object 클래스 - 2
| toString() 메서드
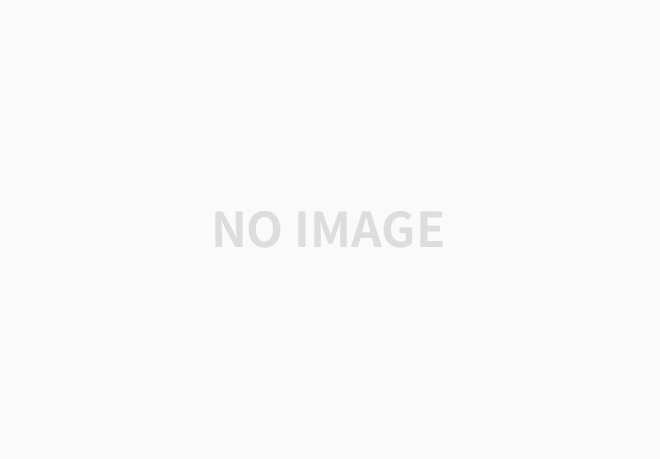
객체 정보를 String 으로 바꿔 사용할 때가 유용하다.
자바 클래스 중에는 이미 정의된 클래스가 많다.
예: String , integer, calendar
많은 클래스가 재정의해서 사용한다.
| equals() 메서드
두 객체의 동일함을 논리적으로 재정의할 수 있음
물리적 동일함: 같은 주소를 가지는 객체
논리적 동일함: 같은 학번의 학생, 같은 주문번호의 주문
물리적으로 다른 메모리에 위치한 객체라도 논리적으로 동일함을 구현하기 위해 사용하는 메서드
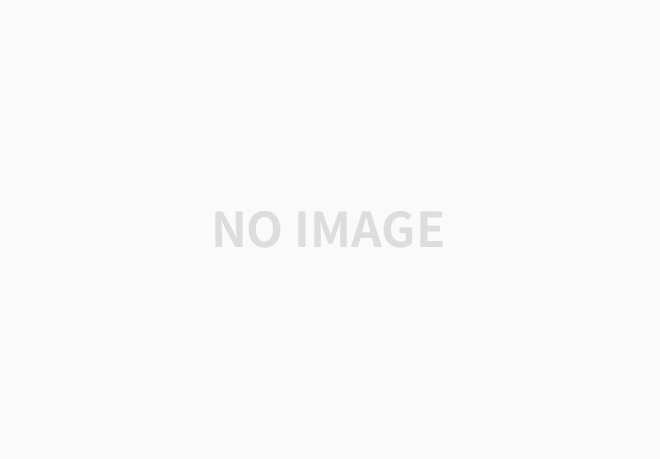
package object;
class Student {
int studentNum;
String studentName;
public Student(int studentNum, String studentName) {
this.studentName = studentName;
this.studentNum = studentNum;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Student) {
Student std = (Student) obj;
return(this.studentNum == std.studentNum);
}
return false;
}
}
public class EqualsTest {
public static void main(String[] args) {
// String str1 = new String("abc");
// String str2 = new String("abc");
//
// System.out.println(str1 == str2);
// System.out.println(str1.equals(str2));
Student Lee = new Student(100, "이순신");
Student Shin = new Student(100, "이순신");
Student Lee2 = Lee;
System.out.println(Lee == Shin);
System.out.println(Lee.equals(Shin));
/*
* false
true
*/
}
}
| hashCode() 메서드
hashCode() 메서드의 반환값: 인스턴스가 저장된 가상 머신의 주소를 10진수로 반환
두 개의 서로 다른 메모리에 위치한 인스턴스가 동일하다는 것은?
논리적으로 동일 : equals() 반환값 true
동일한 hashCode 를 가짐:hashCode()의 반환값이 동일
package object;
class Student {
int studentNum;
String studentName;
public Student(int studentNum, String studentName) {
this.studentName = studentName;
this.studentNum = studentNum;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Student) {
Student std = (Student) obj;
return(this.studentNum == std.studentNum);
}
return false;
}
}
public class EqualsTest {
public static void main(String[] args) {
// String str1 = new String("abc");
// String str2 = new String("abc");
//
// System.out.println(str1 == str2);
// System.out.println(str1.equals(str2));
Student Lee = new Student(100, "이순신");
Student Shin = new Student(100, "이순신");
Student Lee2 = Lee;
System.out.println(Lee.hashCode());
System.out.println(Lee == Shin);
System.out.println(Lee.equals(Shin));
/*
* 2046562095
false
true
*/
}
}
자바 인강이 듣고 싶다면 =>https://bit.ly/3ilMbIO
'스프링, 자바' 카테고리의 다른 글
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 34회차 미션 (0) | 2020.09.12 |
---|---|
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 33회차 미션 (0) | 2020.09.11 |
09. 운영체제 구조 - 사용자 모드와 커널 모드 (0) | 2020.09.09 |
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 31회차 미션 (0) | 2020.09.09 |
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 30회차 미션 (0) | 2020.09.08 |