반응형
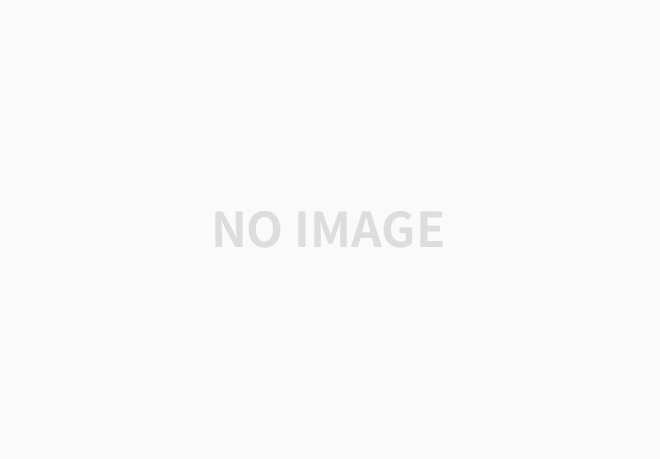
23. ArrayList 사용하기 - 2
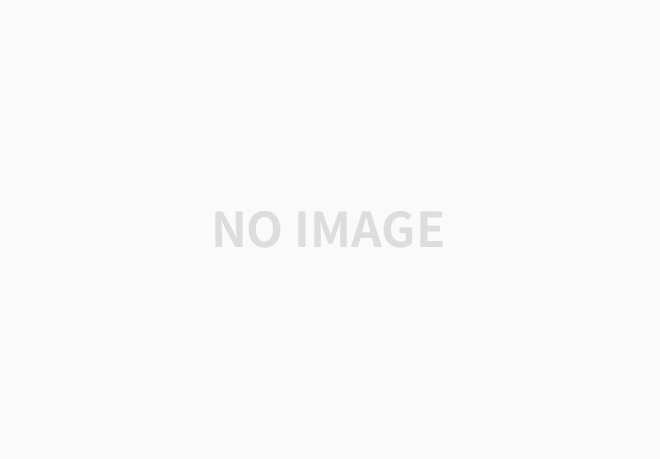
package array;
public class Subject {
private String name;
private int score;
public Subject(String name, int score) {
this.name = name;
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
}
package array;
import java.util.ArrayList;
public class Student {
int studentID;
String studentName;
ArrayList<Subject> subjectList;
public Student(int studentID, String studentName) {
this.studentID = studentID;
this.studentName = studentName;
subjectList = new ArrayList<Subject>();
}
public void addSubject(String name, int score) {
Subject subject = new Subject(name, score);
subjectList.add(subject);
}
public void showStudentInfo() {
int total =0;
for(Subject subject : subjectList) {
total +=subject.getScore();
System.out.println(studentName +"학생의 " +subject.getName()+"과목의 성적은 "+subject.getScore()+"점 입니다.");
}
System.out.println(studentName +"학생의 " +"총과목의 성적은 "+total+"점 입니다.");
}
}
package array;
public class StudentTEst {
public static void main(String[] args) {
Student studentLee = new Student(1001, "Lee");
Student studentKim = new Student(1002, "Kim");
studentLee.addSubject("국어", 100);
studentLee.addSubject("수학", 90);
studentKim.addSubject("수학", 30);
studentKim.addSubject("과학", 100);
studentKim.addSubject("영어", 100);
studentLee.showStudentInfo();
System.out.println("==============");
studentKim.showStudentInfo();
/*
*
* Lee학생의 국어과목의 성적은 100점 입니다.
Lee학생의 수학과목의 성적은 90점 입니다.
Lee학생의 총과목의 성적은 190점 입니다.
==============
Kim학생의 수학과목의 성적은 30점 입니다.
Kim학생의 과학과목의 성적은 100점 입니다.
Kim학생의 영어과목의 성적은 100점 입니다.
Kim학생의 총과목의 성적은 230점 입니다.
*/
}
}
24. 코딩해 보세요
[ 단축키 ] 자동 import : ctrl + shift + o
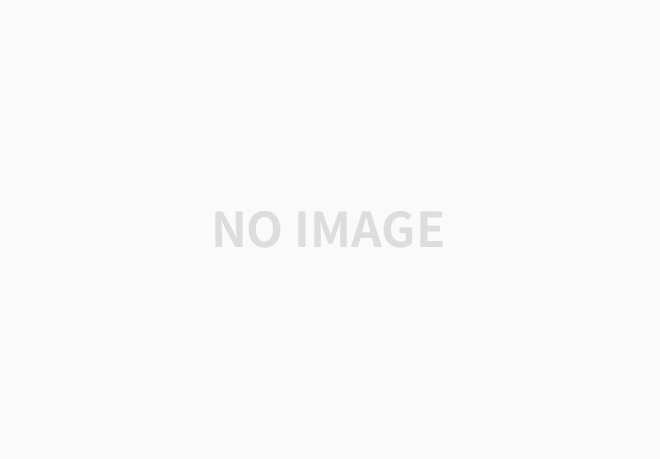
package practice;
public class BookTest {
public static void main(String[] args) {
Student studentLee = new Student("Lee");
Student studentKim =new Student("Kim");
Student studentCho =new Student("Cho");
studentLee.addBook("태백산맥 1");
studentLee.addBook("태백산맥 2");
studentLee.addBook("태백산맥 3");
studentKim.addBook("토지 1");
studentKim.addBook("토지 2");
studentKim.addBook("토지 3");
studentCho.addBook("해리포터 1");
studentCho.addBook("해리포터 2");
studentCho.addBook("해리포터 3");
studentCho.addBook("해리포터 4");
studentCho.addBook("해리포터 5");
studentCho.addBook("해리포터 6");
studentCho.getStudentInfo();
studentLee.getStudentInfo();
studentKim.getStudentInfo();
/*
* Cho 학생이 읽은 책은 : 해리포터 1 해리포터 2 해리포터 3 해리포터 4 해리포터 5 해리포터 6 입니다.
Lee 학생이 읽은 책은 : 태백산맥 1 태백산맥 2 태백산맥 3 입니다.
Kim 학생이 읽은 책은 : 토지 1 토지 2 토지 3 입니다.
*/
}
}
package practice;
public class Book {
private String name;
public Book(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
package practice;
import java.util.ArrayList;
public class Student {
private String myname;
private ArrayList<Book> bookList;
public Student(String name) {
this.myname = name;
bookList = new ArrayList<Book>();
}
public void addBook(String book_name) {
bookList.add(new Book(book_name));
}
public void getStudentInfo() {
System.out.print(this.myname + " 학생이 읽은 책은 : ");
for (Book book_name : bookList) {
System.out.print(book_name.getName()+" ");
}
System.out.println("입니다.");
}
}
자바 인강이 듣고 싶다면 => https://bit.ly/3ilMbIO
반응형
'스프링, 자바' 카테고리의 다른 글
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 24회차 미션 (0) | 2020.09.02 |
---|---|
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 23회차 미션 (0) | 2020.09.01 |
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 21회차 미션 (0) | 2020.08.30 |
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 20회차 미션 (0) | 2020.08.29 |
[패스트캠퍼스 수강 후기] 자바 인강 100% 환급 챌린지 19회차 미션 (0) | 2020.08.28 |